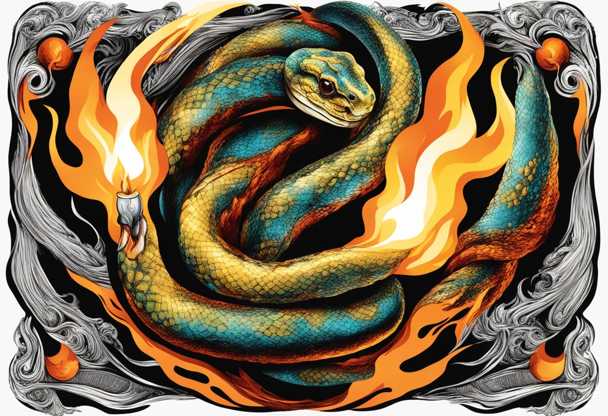
What Exactly is Torch?
Torch, often referred to as PyTorch when discussing its Python implementation, is an open-source machine learning library developed by Facebook’s AI Research lab (FAIR). It provides a flexible and powerful platform for deep learning and neural network projects. At its core, Torch is designed to support tensor computations and build computational graphs, making it an essential tool for data scientists, AI researchers, and developers working on artificial intelligence, computer vision, natural language processing, and more.
Key Features of Torch
– Tensors: At the heart of Torch lies the concept of tensors, which are multi-dimensional arrays. Tensors are fundamental to deep learning as they allow for the efficient handling of large datasets and the execution of complex mathematical operations.
– Autograd: Torch’s automatic differentiation engine, Autograd, plays a critical role in training neural networks. It automatically computes gradients, making backpropagation—a key algorithm in neural network training—easy to implement.
– Dynamic Computational Graphs: Unlike some other deep learning frameworks, Torch supports dynamic computational graphs. This flexibility allows you to change the graph on the fly, making it easier to experiment with different neural network architectures.
– GPU Acceleration: Torch is optimized for running on GPUs, allowing for significant speed improvements in training deep learning models. It supports CUDA (Compute Unified Device Architecture) operations, making it possible to leverage NVIDIA GPUs.
– TorchScript: TorchScript allows developers to transition seamlessly from research to production by providing a way to serialize and optimize models. It bridges the gap between Python development and production deployment.
Step-by-Step Guide to Installing Torch with pip
Installing Torch is a straightforward process, especially with pip, Python’s package installer. Below are the steps to install Torch on your system.
Step 1: Install Python
Before installing Torch, ensure that Python is installed on your system. Torch is compatible with Python versions 3.6 and above. You can download the latest version of Python from the official Python website
Step 2: Create a Virtual Environment (feel free to skip this step if you are overwhelmed)
It’s good practice to create a virtual environment for your Python projects. Virtual environments help manage dependencies and avoid conflicts between different projects.
To create a virtual environment, use the following commands:
python -m venv myenv
Activate the virtual environment:
– On Windows:
myenv\Scripts\activate
– On macOS/Linux:
source myenv/bin/activate
Step 3: Install pip (if not already installed)
Most Python distributions come with pip pre-installed. You can verify if pip is installed by running:
pip –version
If pip is not installed, you can install it using the following command:
python -m ensurepip –upgrade
Step 4: Install Torch
Once pip is set up, you can install Torch by running:
pip install torch torchvision torchaudio
– torch: The core library for tensor computation.
– torchvision: A package that provides access to popular datasets, model architectures, and image transformations.
– torchaudio: A package for working with audio data.
Step 5: Verify Installation
After installation, you can verify that Torch is installed correctly by running a simple Python script:
python
import torch
print(torch.__version__)
This script will print the installed version of Torch.
Example of a Simple Torch Script
Now that Torch is installed, let’s dive into a basic example. The following script demonstrates how to create a simple neural network to classify handwritten digits from the MNIST dataset.
python
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision
import torchvision.transforms as transforms
# Define a simple neural network
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(28*28, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = x.view(-1, 28*28)
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Load the MNIST dataset
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))])
trainset = torchvision.datasets.MNIST(root=’./data’, train=True, download=True, transform=transform)
trainloader = torch.utils.data.DataLoader(trainset, batch_size=64, shuffle=True)
# Initialize the network, loss function, and optimizer
net = SimpleNN()
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(net.parameters(), lr=0.01)
# Train the network
for epoch in range(2):
running_loss = 0.0
for i, data in enumerate(trainloader, 0):
inputs, labels = data
optimizer.zero_grad()
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
if i % 100 == 99:
print(f'[Epoch {epoch + 1}, Batch {i + 1}] loss: {running_loss / 100:.3f}’)
running_loss = 0.0
print(‘Finished Training’)
This script does the following:
1. Defines a simple neural network with two fully connected layers.
2. Loads the MNIST dataset using torchvision’s dataset utilities.
3. Trains the network on the MNIST data for two epochs.
4. Prints the loss every 100 batches to monitor training progress.
What Can Torch Do Now?
Torch is a versatile tool that supports a wide range of applications in AI and deep learning. Some of its current capabilities include:
1. Image Classification and Object Detection
Torch, through the use of pre-trained models and libraries like `torchvision`, makes it straightforward to perform image classification and object detection tasks. Popular models like ResNet, VGG, and YOLO can be easily implemented with Torch.
2. Natural Language Processing (NLP)
Torch is widely used in NLP tasks such as sentiment analysis, text generation, machine translation, and chatbots. Libraries like Hugging Face’s Transformers are built on top of Torch and provide pre-trained models like BERT and GPT, which can be fine-tuned for various NLP tasks.
3. Generative Models
Torch is instrumental in developing generative models such as Generative Adversarial Networks (GANs) and Variational Autoencoders (VAEs). These models are used in generating realistic images, videos, and even music.
4. Reinforcement Learning
Torch is used in reinforcement learning, where agents learn to make decisions by interacting with an environment. Libraries like Stable Baselines are built on Torch and provide implementations of popular reinforcement learning algorithms.
5. Scientific Computing
Beyond AI, Torch’s tensor operations make it suitable for scientific computing tasks, including numerical simulations, data analysis, and solving differential equations.
What Does the Future Hold for Torch?
As artificial intelligence and machine learning continue to evolve, the future of Torch looks promising. Its flexibility, ease of use, and extensive community support position it as a key player in the AI landscape. Here are some potential future directions for Torch:
1. Integration with Quantum Computing
As quantum computing matures, there’s a possibility that Torch could integrate with quantum computing frameworks. This would open up new possibilities for solving complex problems that are currently infeasible with classical computing.
2. Expansion into Edge Computing
With the rise of IoT and edge computing, there’s a growing demand for running AI models on edge devices with limited computational resources. Torch’s lightweight and efficient nature make it a good candidate for deployment on such devices.
3. Greater Adoption in Industry
Torch has already seen significant adoption in both academia and industry. As more companies invest in AI, Torch could become the go-to framework for developing and deploying AI solutions in production environments.
4. Enhanced Support for Multi-Modal AI
The future of AI is multi-modal, combining text, images, audio, and video. Torch’s ability to handle various data types positions it well for advancements in multi-modal AI, enabling the development of more sophisticated and human-like AI systems.
Will Torch Be Replaced by Machine Learning and AI, or Will It Aid in Accelerating AI Technology?
Torch is a tool for machine learning and AI, so the question of whether it will be replaced by these technologies is somewhat misplaced. Instead, Torch is likely to play a crucial role in advancing AI technology. Here’s why:
1. Continued Evolution
Torch is continuously evolving, with frequent updates and contributions from the open-source community. As new AI techniques and algorithms emerge, Torch is likely to incorporate them, ensuring it remains at the forefront of AI development.
2. Accelerating Research and Development
Torch’s user-friendly interface and comprehensive documentation make it easier for researchers and developers to experiment with new ideas. This accelerates the pace of AI research and the development of new applications.
3. Bridging the Gap Between Research and Production
With features like TorchScript, Torch makes it easier to transition models from the research phase to production deployment. This seamless transition is crucial for scaling AI solutions in real-world applications.
4. Synergy with Other Technologies
Torch’s modular design allows it to work synergistically with other AI technologies, such as TensorFlow, Keras, and ONNX (Open Neural Network Exchange). This interoperability ensures that Torch will continue to be relevant as the AI ecosystem evolves.
Conclusion: What Does the Future Hold for Using Torch in Python?
As we look to the future, several questions arise about the trajectory of Torch and its
1. What innovations will Torch introduce in the coming years, and how will they reshape AI and machine learning development?
2. How will Torch continue to evolve alongside Python, and what new libraries or features might emerge from their integration?
3. Will Torch maintain its relevance in the face of growing competition from other AI frameworks, or will it be surpassed by newer technologies?
4. As Torch becomes more sophisticated, how will it influence the accessibility of AI tools for non-experts and hobbyists?
5. What role will Torch play in the development of ethical AI, and how might it contribute to addressing biases or other challenges in AI models?
6. How will Torch’s advancements affect the pace and scale of AI adoption across various industries, and which sectors will be most impacted?
7. Could Torch’s integration with quantum computing or other emerging technologies lead to breakthroughs in AI capabilities, and if so, what might those look like?
8. Will Torch eventually become the standard for AI development in Python, or could a new framework disrupt its dominance?
9. How might the growing adoption of Torch influence the direction of AI research and the types of problems researchers choose to tackle?
10. In what ways will the broader AI community collaborate to enhance Torch, and what impact will this have on the global AI landscape?
These questions can stimulate further reflection on the potential future of Torch in Python and its broader implications for AI development and adoption. Feel free to post your comments below.
Leave a Reply